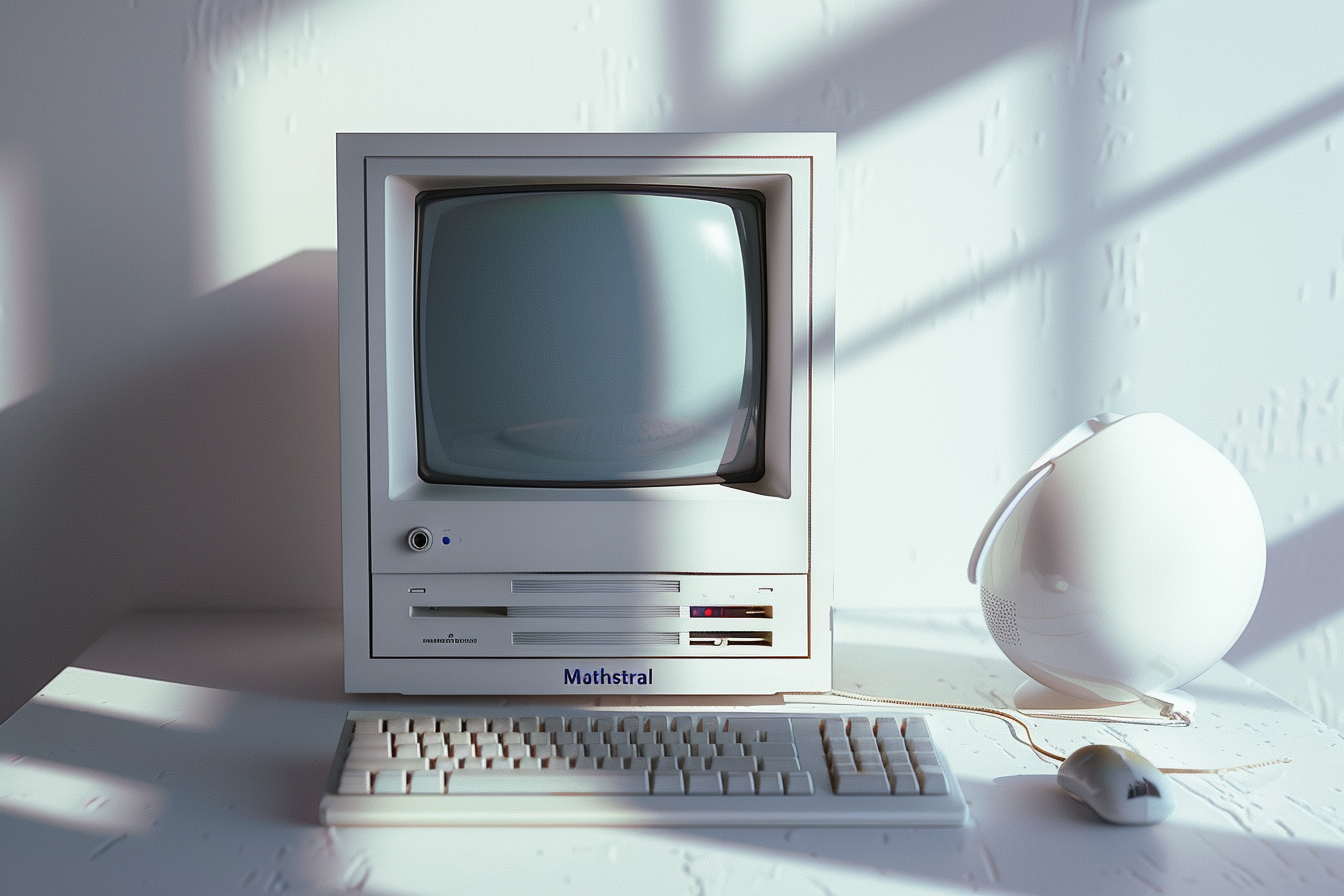
Large Language Models (LLMs) have revolutionized the field of artificial intelligence, enabling machines to understand and generate human-like text with unprecedented accuracy. However, one of the key challenges in deploying LLMs for real-world applications is their inherent lack of memory. By default, LLMs are stateless, treating each input as an isolated query without any context from previous interactions. This limitation can lead to inconsistent and disjointed conversations, especially in chatbot applications where maintaining context is crucial.
To address this challenge, researchers and developers have been working on various memory mechanisms for LLMs. These mechanisms aim to provide LLMs with the ability to retain and recall information from past interactions, enabling more coherent and context-aware conversations. In this article, we'll explore the concept of LLM memory, its importance, different types of memory implementations, and their practical applications.
You can easily create AI workflows with Anakin AI without any coding knowledge. Connect to LLM APIs such as: GPT-4, Claude 3.5 Sonnet, Uncensored Dolphin-Mixtral, Stable Diffusion, DALLE, Web Scraping.... into One Workflow!
Forget about complicated coding, automate your madane work with Anakin AI!
For a limited time, you can also use Google Gemini 1.5 and Stable Diffusion for Free!
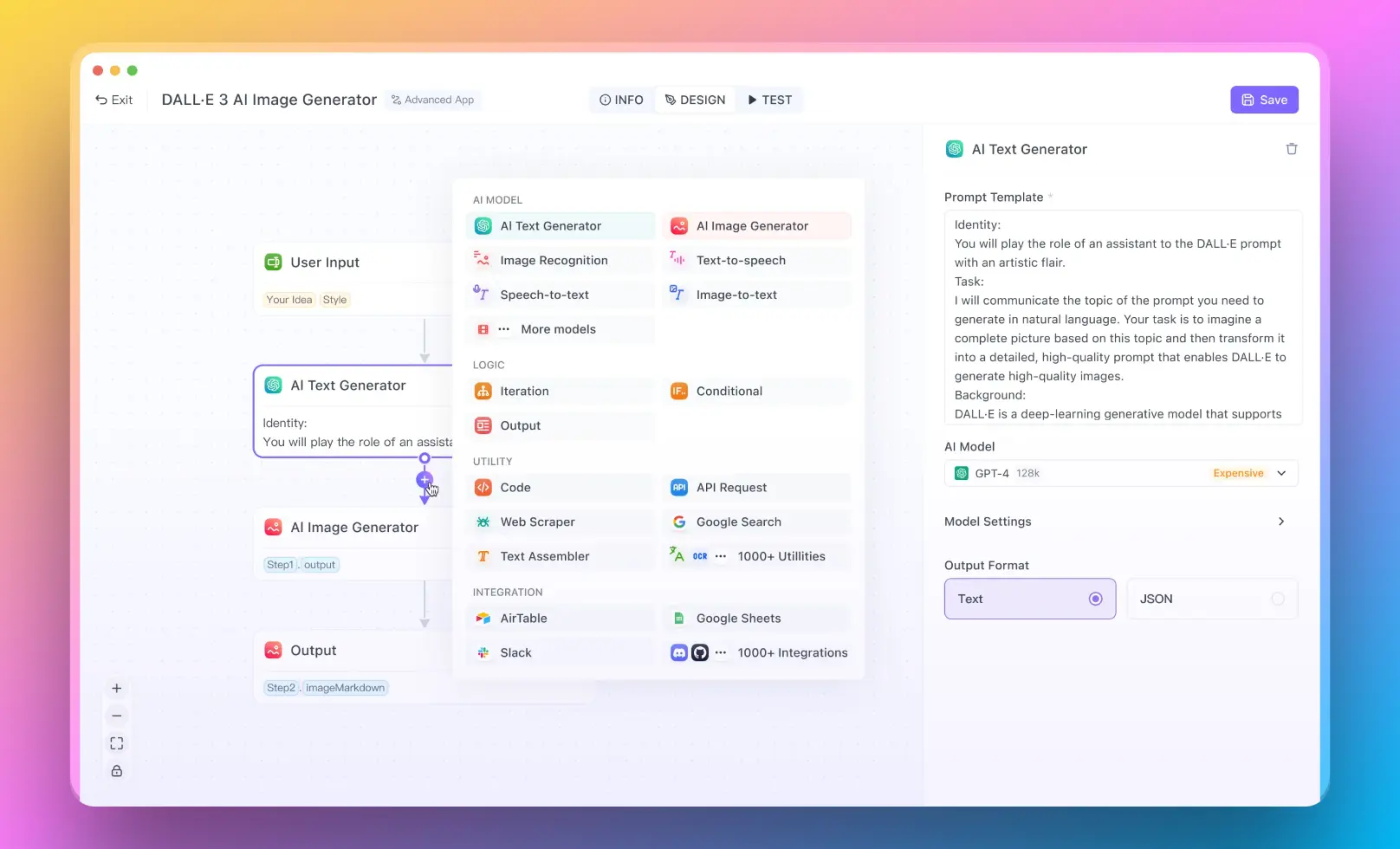
The Importance of Memory in LLMs
Memory in LLMs serves several critical functions:
- Contextual Understanding: It allows the model to understand and respond to queries in the context of previous interactions.
- Personalization: Memory enables the LLM to remember user preferences and tailor responses accordingly.
- Long-term Learning: With memory, LLMs can accumulate knowledge over time, improving their performance in specific domains.
- Consistency: Memory helps maintain consistent information across multiple interactions, avoiding contradictions.
Without memory, LLMs would struggle to maintain coherent conversations or provide personalized experiences, limiting their usefulness in many real-world applications.
Types of LLM Memory
There are several approaches to implementing memory in LLMs, each with its own strengths and use cases:
1. Short-term Memory (Conversation Buffer)
Short-term memory, often implemented as a conversation buffer, is the simplest form of LLM memory. It involves storing recent interactions in a buffer and including them in the context for subsequent queries.
Implementation Example:
from langchain.memory import ConversationBufferMemory
from langchain.llms import OpenAI
from langchain.chains import ConversationChain
llm = OpenAI(temperature=0)
memory = ConversationBufferMemory()
conversation = ConversationChain(
llm=llm,
memory=memory,
verbose=True
)
conversation.predict(input="Hi, I'm Alice.")
conversation.predict(input="What's my name?")
In this example, the ConversationBufferMemory stores the entire conversation history, allowing the LLM to recall previous interactions.
2. Summary Memory
Summary memory involves periodically summarizing the conversation and storing only the summary instead of the entire history. This approach helps manage token limits while retaining key information.
Implementation Example:
from langchain.memory import ConversationSummaryMemory
memory = ConversationSummaryMemory(llm=llm)
conversation = ConversationChain(
llm=llm,
memory=memory,
verbose=True
)
conversation.predict(input="Hi, I'm Bob. I like playing tennis.")
conversation.predict(input="What sport do I like?")
ConversationSummaryMemory uses the LLM to generate summaries of the conversation, which are then used as context for future interactions.
3. Vector Store Memory
Vector store memory involves converting conversations into numerical representations (embeddings) and storing them in a vector database. This allows for efficient similarity-based retrieval of relevant past interactions.
Implementation Example:
from langchain.embeddings import OpenAIEmbeddings
from langchain.vectorstores import Chroma
from langchain.memory import VectorStoreRetrieverMemory
embeddings = OpenAIEmbeddings()
vectorstore = Chroma("langchain_store", embeddings.embed_query)
memory = VectorStoreRetrieverMemory(retriever=vectorstore.as_retriever())
conversation = ConversationChain(
llm=llm,
memory=memory,
verbose=True
)
conversation.predict(input="My favorite color is blue.")
conversation.predict(input="What's my favorite color?")
This approach allows for more flexible and scalable memory retrieval, especially for large-scale applications.
4. Hierarchical Memory
Hierarchical memory systems attempt to mimic human memory organization by creating a structured hierarchy of memories. This can involve short-term buffers, long-term storage, and intermediate summaries.
Conceptual Implementation:
class HierarchicalMemory:
def __init__(self):
self.short_term_buffer = []
self.long_term_storage = {}
self.summaries = {}
def add_memory(self, memory):
self.short_term_buffer.append(memory)
if len(self.short_term_buffer) > 10:
self.consolidate_memories()
def consolidate_memories(self):
summary = self.generate_summary(self.short_term_buffer)
topic = self.identify_topic(summary)
if topic in self.long_term_storage:
self.long_term_storage[topic].append(summary)
else:
self.long_term_storage[topic] = [summary]
self.short_term_buffer = []
def retrieve_memories(self, query):
relevant_topic = self.identify_topic(query)
return self.long_term_storage.get(relevant_topic, [])
def generate_summary(self, memories):
# Use LLM to generate summary
pass
def identify_topic(self, text):
# Use LLM to identify topic
pass
This hierarchical approach allows for more nuanced memory management, potentially leading to more human-like recall and understanding.
Challenges in Implementing LLM Memory
While memory mechanisms greatly enhance the capabilities of LLMs, they also introduce several challenges:
Token Limits: Most LLMs have a maximum context length, limiting the amount of memory that can be included in each interaction.
Relevance: Determining which memories are relevant to the current context is a complex task that can significantly impact the quality of responses.
Privacy and Security: Storing user interactions raises important privacy and security concerns, especially for sensitive applications.
Scalability: As conversations grow longer and user bases expand, efficiently managing and retrieving memories becomes increasingly challenging.
Consistency: Ensuring that memories remain consistent over time and across different interactions is crucial for maintaining the illusion of a coherent AI personality.
Advanced Memory Techniques
To address these challenges, researchers are exploring more sophisticated memory techniques:
Episodic Memory
Inspired by human cognition, episodic memory aims to store and retrieve specific experiences or events. This can be particularly useful for personalized AI assistants that need to recall user-specific information.
class EpisodicMemory:
def __init__(self):
self.episodes = []
def add_episode(self, episode):
self.episodes.append({
'timestamp': time.time(),
'content': episode
})
def retrieve_relevant_episodes(self, query):
relevant_episodes = []
for episode in self.episodes:
if self.is_relevant(query, episode['content']):
relevant_episodes.append(episode)
return relevant_episodes
def is_relevant(self, query, episode):
# Use similarity measures or LLM to determine relevance
pass
Attention-based Memory
This approach uses attention mechanisms to selectively focus on the most relevant parts of the memory when generating responses.
class AttentionMemory:
def __init__(self, embedding_model):
self.memories = []
self.embedding_model = embedding_model
def add_memory(self, memory):
embedding = self.embedding_model.embed(memory)
self.memories.append({
'content': memory,
'embedding': embedding
})
def retrieve_memories(self, query):
query_embedding = self.embedding_model.embed(query)
attention_scores = self.compute_attention(query_embedding)
return self.apply_attention(attention_scores)
def compute_attention(self, query_embedding):
scores = []
for memory in self.memories:
similarity = self.cosine_similarity(query_embedding, memory['embedding'])
scores.append(similarity)
return self.softmax(scores)
def apply_attention(self, attention_scores):
weighted_memories = []
for score, memory in zip(attention_scores, self.memories):
weighted_memories.append({
'content': memory['content'],
'weight': score
})
return weighted_memories
def cosine_similarity(self, a, b):
# Compute cosine similarity between vectors
pass
def softmax(self, scores):
# Compute softmax of scores
pass
Memory Compression
To manage token limits, memory compression techniques can be employed to reduce the size of stored memories while retaining essential information.
class CompressedMemory:
def __init__(self, llm):
self.memories = []
self.llm = llm
def add_memory(self, memory):
compressed = self.compress(memory)
self.memories.append(compressed)
def compress(self, memory):
prompt = f"Compress the following information into a concise summary:\n{memory}"
return self.llm.generate(prompt)
def decompress(self, compressed_memory):
prompt = f"Expand the following compressed information:\n{compressed_memory}"
return self.llm.generate(prompt)
def retrieve_memories(self, query):
relevant_memories = self.find_relevant_memories(query)
return [self.decompress(memory) for memory in relevant_memories]
def find_relevant_memories(self, query):
# Implement relevance scoring and retrieval
pass
Future Directions in LLM Memory
As research in LLM memory continues to advance, several exciting directions are emerging:
Adaptive Memory Management: Developing systems that can dynamically adjust their memory strategies based on the conversation context and available resources.
Multi-modal Memory: Integrating memory systems that can handle not just text, but also images, audio, and other forms of data.
Federated Memory: Exploring ways to share and aggregate memories across multiple instances of an LLM while preserving privacy and security.
Causal Memory: Implementing memory systems that can understand and reason about cause-and-effect relationships in stored information.
Memory Editing and Forgetting: Developing mechanisms for selectively editing or removing memories to maintain consistency and manage storage limitations.
Conclusion
Memory mechanisms are crucial for enhancing the capabilities of Large Language Models, enabling them to maintain context, personalize responses, and provide more coherent and engaging interactions. From simple conversation buffers to sophisticated hierarchical and episodic memory systems, the field of LLM memory is rapidly evolving.
As we continue to push the boundaries of what's possible with LLMs, memory will play an increasingly important role in creating AI systems that can truly understand and engage with users in meaningful ways. The challenges are significant, but the potential rewards – in terms of more capable, personalized, and human-like AI interactions – are immense.
By combining advanced memory techniques with the already impressive capabilities of LLMs, we are moving closer to creating AI systems that can not only process and generate language but also learn, remember, and grow from their interactions. This evolution promises to unlock new applications and possibilities across a wide range of fields, from customer service and education to healthcare and scientific research.
As we look to the future, the continued development of LLM memory systems will be crucial in realizing the full potential of artificial intelligence, bringing us ever closer to the goal of creating truly intelligent and adaptable AI assistants that can understand, remember, and engage with us in increasingly natural and meaningful ways.
FAQs
Q: How does the Memory Stream in LLMs work?
A: The Memory Stream in LLMs works by storing and retrieving past interactions or relevant information, allowing the model to maintain context across conversations. It can be implemented through various methods such as conversation buffers, summaries, or vector stores.
Q: What are some practical examples of using memory abstraction layers in LLMs?
A: Practical examples include chatbots that remember user preferences, customer support systems that recall previous interactions, and personalized AI assistants that maintain context across multiple sessions.
Q: Can you provide a sample code snippet for implementing memory in a conversational AI?
A: Here's a basic example using LangChain's ConversationBufferMemory:
from langchain.memory import ConversationBufferMemory
from langchain.llms import OpenAI
from langchain.chains import ConversationChain
llm = OpenAI(temperature=0)
memory = ConversationBufferMemory()
conversation = ConversationChain(
llm=llm,
memory=memory,
verbose=True
)
conversation.predict(input="Hi, I'm Alice.")
conversation.predict(input="What's my name?")
Q: How does the concept of Reflection enhance the Memory Stream in LLMs?
A: Reflection allows LLMs to analyze and summarize their own thought processes and memories, enabling more efficient storage and retrieval of relevant information. This can lead to improved context understanding and more coherent long-term interactions.
Q: What are the main challenges in developing memory systems for LLMs?
A: The main challenges include managing token limits, determining relevance of stored information, ensuring privacy and security, maintaining scalability for large-scale applications, and preserving consistency across interactions.
from Anakin Blog http://anakin.ai/blog/llm-memory/
via IFTTT
No comments:
Post a Comment